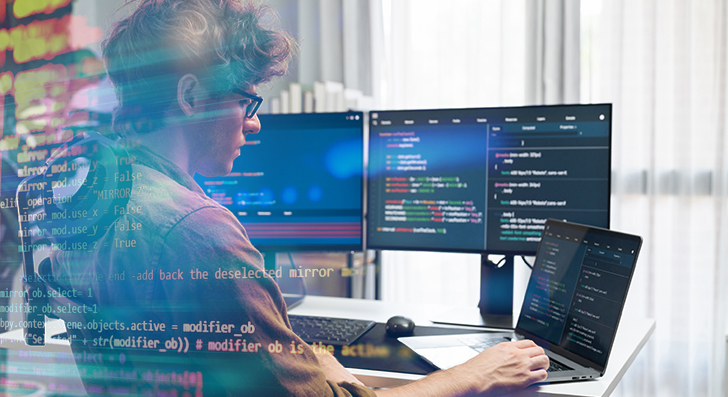
Scalability implies your software can take care of progress—much more users, additional knowledge, and even more visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and anxiety afterwards. Below’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on afterwards—it should be aspect of one's system from the beginning. Quite a few programs are unsuccessful once they improve quick for the reason that the original design and style can’t tackle the extra load. For a developer, you have to Assume early about how your technique will behave stressed.
Begin by coming up with your architecture to be versatile. Stay clear of monolithic codebases exactly where anything is tightly related. As an alternative, use modular style and design or microservices. These patterns break your app into scaled-down, independent elements. Just about every module or company can scale on its own without having influencing The complete method.
Also, think of your databases from working day one. Will it require to deal with 1,000,000 people or just a hundred? Choose the proper variety—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them still.
A further important stage is to prevent hardcoding assumptions. Don’t compose code that only operates under present situations. Think of what would come about If the consumer base doubled tomorrow. Would your application crash? Would the databases slow down?
Use structure styles that aid scaling, like message queues or occasion-driven methods. These assist your app cope with extra requests with no getting overloaded.
When you build with scalability in mind, you're not just planning for achievement—you are lowering foreseeable future problems. A well-prepared technique is easier to take care of, adapt, and expand. It’s far better to organize early than to rebuild later.
Use the Right Databases
Selecting the correct databases is a critical A part of building scalable purposes. Not all databases are designed the same, and using the wrong one can slow you down or perhaps induce failures as your app grows.
Begin by knowing your data. Could it be hugely structured, like rows within a table? If yes, a relational databases like PostgreSQL or MySQL is a great match. These are generally robust with relationships, transactions, and consistency. Additionally they aid scaling tactics like study replicas, indexing, and partitioning to take care of far more visitors and facts.
Should your facts is more adaptable—like user action logs, item catalogs, or paperwork—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with substantial volumes of unstructured or semi-structured information and might scale horizontally more very easily.
Also, take into consideration your go through and generate patterns. Will you be doing a great deal of reads with much less writes? Use caching and read replicas. Have you been managing a heavy compose load? Check into databases that can deal with substantial generate throughput, or perhaps function-dependent details storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to think ahead. You may not want Innovative scaling capabilities now, but selecting a database that supports them implies you gained’t need to have to modify afterwards.
Use indexing to hurry up queries. Stay clear of avoidable joins. Normalize or denormalize your data based on your accessibility patterns. And usually check database efficiency while you expand.
In a nutshell, the correct database is determined by your app’s structure, speed needs, and how you expect it to mature. Choose time to select correctly—it’ll help save a great deal of problems later.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you build economical logic from the beginning.
Commence by creating clean up, uncomplicated code. Keep away from repeating logic and remove anything unwanted. Don’t select the most complicated Alternative if an easy a single works. Maintain your functions shorter, centered, and easy to check. Use profiling resources to find bottlenecks—destinations in which your code takes far too lengthy to operate or makes use of too much memory.
Upcoming, take a look at your databases queries. These frequently gradual items down much more than the code by itself. Ensure Each and every question only asks for the information you truly require. Prevent Choose *, which fetches anything, and as an alternative pick out particular fields. Use indexes to hurry up lookups. And avoid carrying out a lot of joins, Specifically throughout big tables.
When you notice precisely the same details becoming asked for many times, use caching. Shop the outcome quickly using equipment like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. As opposed to updating a row one by one, update them in teams. This cuts down on overhead and will make your application much more effective.
Remember to take a look at with significant datasets. Code and queries that work good with one hundred information may possibly crash if they have to take care of one million.
To put it briefly, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when required. These measures support your software keep clean and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's got to manage additional people plus much more targeted visitors. If all the things goes as a result of a person server, it will immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment aid keep your application speedy, secure, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server doing many of the get the job done, the load balancer routes end users to diverse servers depending on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to setup.
Caching is about storing data quickly so it may be reused quickly. When people request the same facts once again—like a product site or even a profile—you don’t need to fetch it with the database when. You'll be able to provide it through the cache.
There are two prevalent varieties of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static data files close to the consumer.
Caching reduces database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve generally. And usually ensure that your cache is updated when knowledge does improve.
In brief, load balancing and caching are uncomplicated but potent instruments. Alongside one another, they help your app cope with much more end users, continue to be quick, and Recuperate from challenges. If you plan to expand, you require both.
Use Cloud and Container Resources
To develop scalable purposes, you need resources that allow your application mature easily. That’s in which cloud platforms and containers can be found in. They provide you adaptability, reduce setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t need to acquire hardware or guess foreseeable future ability. When website traffic boosts, you could increase extra resources with just some clicks or automatically using auto-scaling. When traffic drops, you can scale down to save money.
These platforms also offer products and services like managed databases, storage, load balancing, and security applications. You could deal with making your application as an alternative to controlling infrastructure.
Containers are An additional important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it quick to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
When your application works by using several containers, tools like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You could update or scale elements independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment usually means it is possible to scale fast, deploy quickly, and recover speedily when problems come about. If you would like your application to mature without having restrictions, commence working with these tools early. They preserve time, cut down threat, and assist you stay focused on making, not fixing.
Check Anything
In the event you don’t keep an eye on your software, you won’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location problems early, and make far better selections as your application grows. It’s a key A part of constructing scalable devices.
Start by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this facts.
Don’t just observe your servers—observe your application too. Keep an eye on how long it takes for customers to load webpages, how often mistakes occur, and exactly where they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you check here see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes above a Restrict or simply a assistance goes down, it is best to get notified promptly. This allows you take care of difficulties rapid, typically just before customers even discover.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again just before it causes serious hurt.
As your app grows, targeted visitors and knowledge boost. Without checking, you’ll skip indications of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In brief, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it really works effectively, even stressed.
Ultimate Views
Scalability isn’t just for major businesses. Even smaller apps need to have a strong foundation. By building very carefully, optimizing sensibly, and using the appropriate applications, it is possible to Establish apps that increase smoothly without having breaking stressed. Start tiny, Imagine large, and Create good.